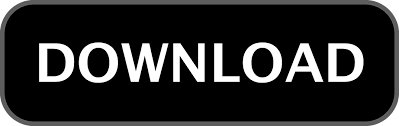

The retry method accepts two arguments: the number of times the request should be attempted and the number of milliseconds that Laravel should wait in between attempts: $response = Http::retry(3, 100)->post(.) If you would like HTTP client to automatically retry the request if a client or server error occurs, you may use the retry method. If the given timeout is exceeded, an instance of Illuminate\Http\Client\ConnectionException will be thrown. The timeout method may be used to specify the maximum number of seconds to wait for a response: $response = Http::timeout(3)->get(.) If you would like to quickly add an Authorization bearer token header to the request, you may use the withToken method: $response = Http::withToken('token')->post(.) Timeout

$response = Http::withDigestAuth(' ', 'secret')->post(.) Bearer Tokens $response = Http::withBasicAuth(' ', 'secret')->post(.) You may specify basic and digest authentication credentials using the withBasicAuth and withDigestAuth methods, respectively: // Basic authentication. This withHeaders method accepts an array of key / value pairs: $response = Http::withHeaders([
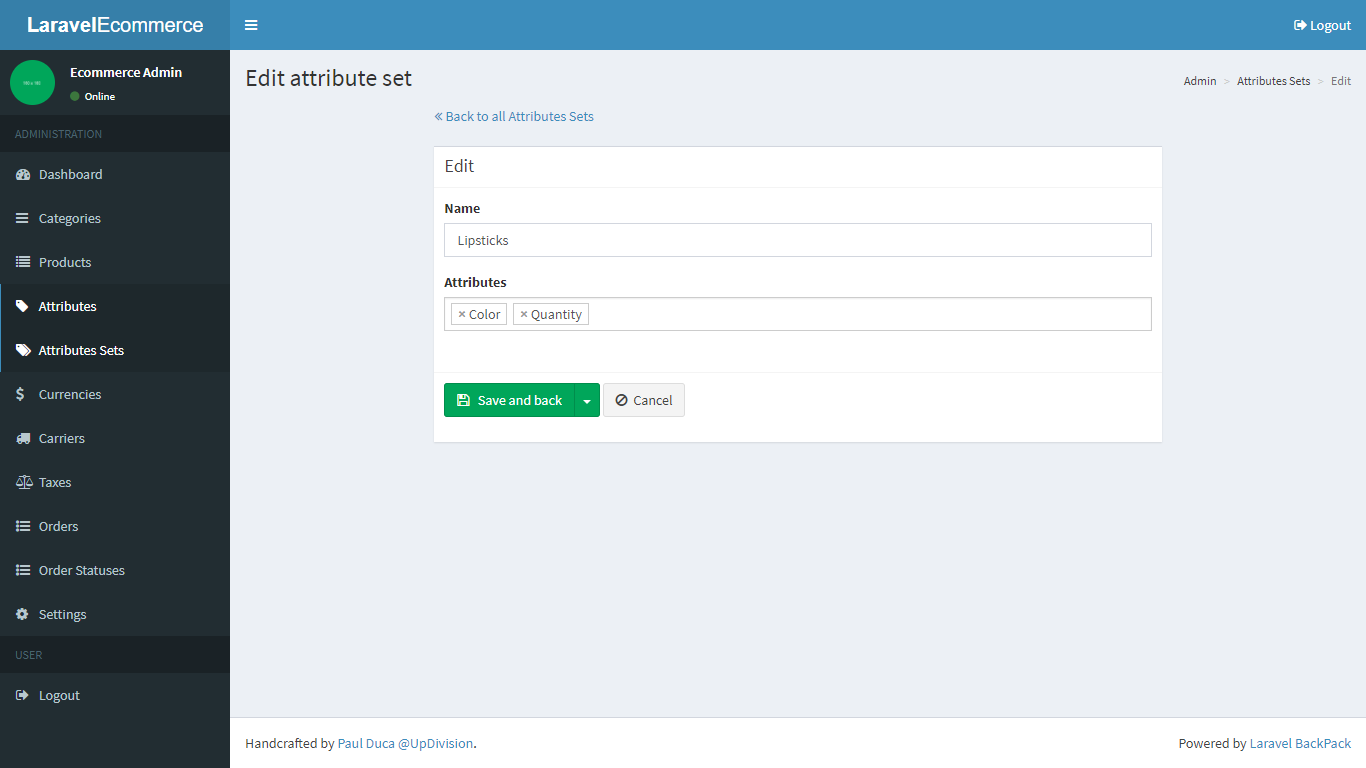
Headers may be added to requests using the withHeaders method. Instead of passing the raw contents of a file, you may also pass a stream resource: $photo = fopen('photo.jpg', 'r') 'attachment', file_get_contents('photo.jpg'), 'photo.jpg' Optionally, you may provide a third argument which will be considered the file's filename: $response = Http::attach( This method accepts the name of the file and its contents. If you would like to send files as multi-part requests, you should call the attach method before making your request. You may use the withBody method if you would like to provide a raw request body when making a request: $response = Http::withBody( If you would like to send data using the application/x-www-form-urlencoded content type, you should call the asForm method before making your request: $response = Http::asForm()->post('', [ When making GET requests, you may either append a query string to the URL directly or pass an array of key / value pairs as the second argument to the get method: $response = Http::get('', [ By default, data will be sent using the application/json content type: $response = Http::post('', [ So, these methods accept an array of data as their second argument.
#LARAVEL HTTP CLIENT PATCH#
Of course, it is common when using POST, PUT, and PATCH to send additional data with your request. The Illuminate\Http\Client\Response object also implements the PHP ArrayAccess interface, allowing you to access JSON response data directly on the response: return Http::get('') Request Data The get method returns an instance of Illuminate\Http\Client\Response, which provides a variety of methods that may be used to inspect the response: $response->body() : string
#LARAVEL HTTP CLIENT HOW TO#
First, let's examine how to make a basic GET request: use Illuminate\Support\Facades\Http To make requests, you may use the get, post, put, patch, and delete methods. By default, Laravel automatically includes this dependency: composer require guzzlehttp/guzzle Making Requests Laravel's wrapper around Guzzle is focused on its most common use cases and a wonderful developer experience.īefore getting started, you should ensure that you have installed the Guzzle package as a dependency of your application. Try it like this to return the response as a String.Laravel provides an expressive, minimal API around the Guzzle HTTP client, allowing you to quickly make outgoing HTTP requests to communicate with other web applications.
#LARAVEL HTTP CLIENT FULL#
Remote URL * Specify the full URL of the remote file Check if gzip is enabled3. To automate this process, I developed an online gzip compression test that tells you whether the server is configured correctly and displays all HTTP response headers. If gzip is enabled, the server should return the Content-Encoding: gzip header.
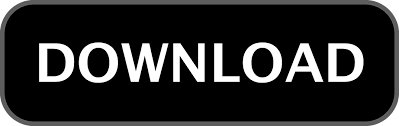